令人费解的AppKit
脱离nib 和 storyboard 开发方式
nib和storyboard 千好万好。但在多人开发模式下,修改同一份storyborad,产生的冲突简直是灾难。
干掉项目中info.plist
关于Main.storyboard
的设置。在main.m
中手动绑定AppDelegate
1 2 3 4 5 6 7
| #import <Cocoa/Cocoa.h> #import "AppDelegate.h" int main(int argc, const char * argv[]) { AppDelegate *app = [[AppDelegate alloc] init]; [NSApplication sharedApplication].delegate = app; return NSApplicationMain(argc, argv); }
|
按照iOS
的开发经验,Window
下要放置一个ViewController
。需要注意的是重写loadview
方法不要调用super
1
| Instantiates a view from a nib file and sets the value of the view property.
|
super
会去加载同名的nib
文件。正确的纯编码方式是不调用父类方法,再自己设置一个NSView
即可。
1 2 3 4 5
| - (void)loadView{ self.view = [[NSView alloc] initWithFrame:NSMakeRect(0, 0, iCleanMainWindowWidth, iCleanMainWindowHeight)]; self.view.wantsLayer = YES; self.view.layer.backgroundColor = [NSColor brownColor].CGColor; }
|
NSTableView
编码使用NSTableView
的话,要配置使用NSScorllView
和 NSTableColumn
。 具体实践是:
1 2 3 4 5 6 7 8 9 10 11 12 13
| _scrollView = [[NSScrollView alloc] initWithFrame:NSMakeRect(iCleanMainWindowWidth - 400, 0, 400, iCleanMainWindowHeight)]; _scrollView.hasVerticalScroller = _scrollView.hasVerticalRuler = YES; _scrollView.hasHorizontalScroller = _scrollView.hasHorizontalRuler = NO; _tableView = [[NSTableView alloc] initWithFrame:_scrollView.bounds]; _tableView.delegate = self; _tableView.dataSource = self; _tableView.headerView = nil; _scrollView.contentView.documentView = _tableView; NSTableColumn *col = [[NSTableColumn alloc] initWithIdentifier:@"col1"]; col.minWidth = 400; [_tableView addTableColumn:col];
|
其中NSTableDataSource
和 NSTableViewDelegate
虽然都是optional
的办法。但仍需要实现下面的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| #pragma mark - NSTableViewDelegate - (NSView *)tableView:(NSTableView *)tableView viewForTableColumn:(NSTableColumn *)tableColumn row:(NSInteger)row{ NSTextField *cell = [tableView makeViewWithIdentifier:@"cellReuseId" owner:self]; if(!cell){ cell = [NSTextField labelWithString:@""]; cell.identifier = @"cellReuseId"; } NSMutableAttributedString *str = [[NSMutableAttributedString alloc] initWithString:[NSString stringWithFormat:@"%@ \n%@",self.data[row].path.lastPathComponent,self.data[row].formatSize] attributes:@{NSForegroundColorAttributeName:[NSColor blackColor]}]; cell.placeholderAttributedString = str; return cell; }
- (CGFloat)tableView:(NSTableView *)tableView heightOfRow:(NSInteger)row{ return 50.f; }
- (void)tableViewSelectionDidChange:(NSNotification *)notification{ NSInteger row = [[notification object] selectedRow]; NSLog(@"%ld",row); }
#pragma mark - NSTableViewDataSource - (NSInteger)numberOfRowsInTableView:(NSTableView *)tableView{ return self.data.count; }
|
注意是 AppKit
里面是没有Label
控件的,我这是使用的是NSTextField
。就是输入框了,借助placeholderString
属性能实现文本展示的效果。
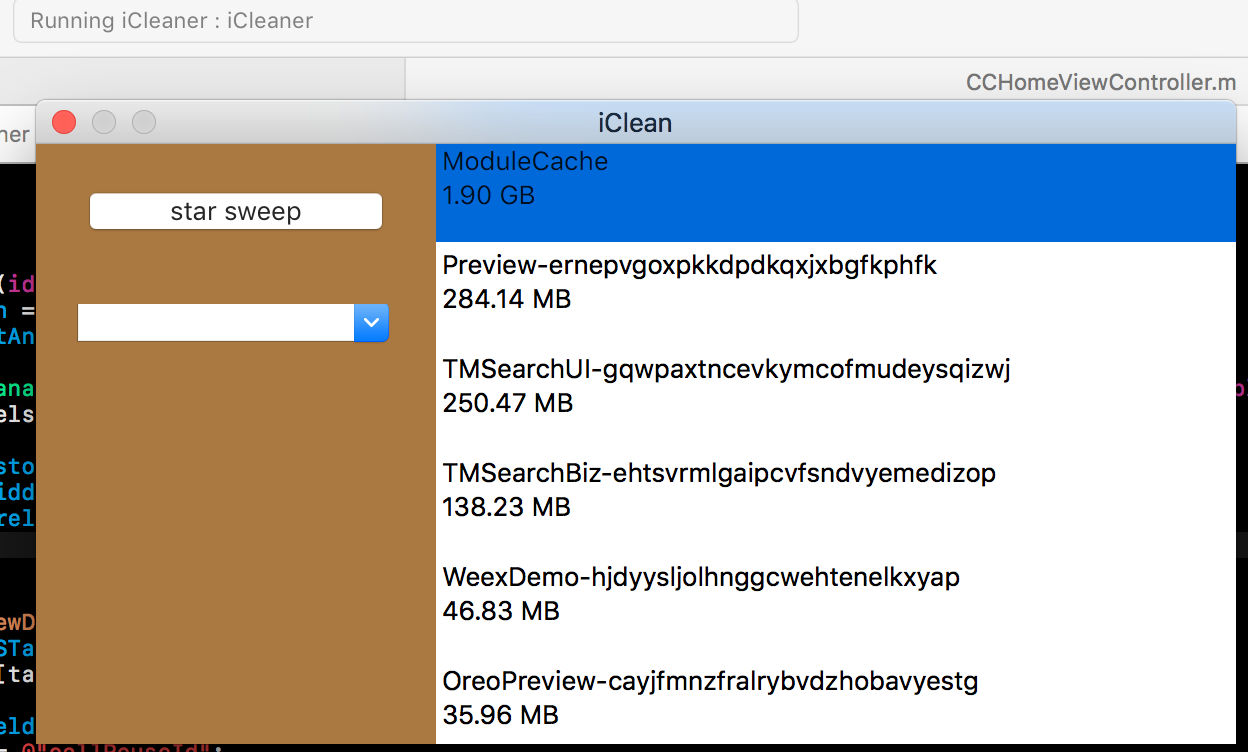